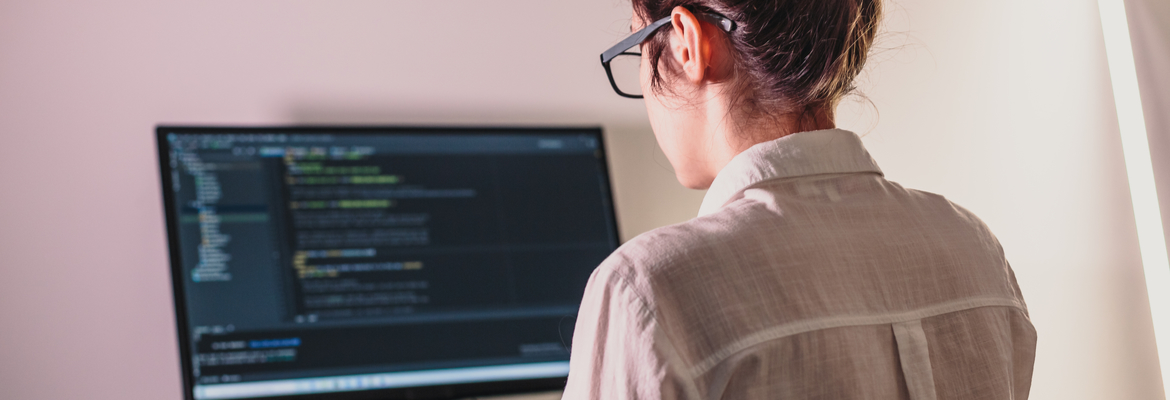
Most people who create applications in Salesforce have a fun time doing it; we get to use a lot of cool technology and make seemingly miraculous things happen. If you are reading this, chances are that you have had at least one moment when somebody asked you, “Whoa, how did you do THAT?”
However, we all know that business rules evolve quickly and human memories are short. This means that when, six months from now, you are asked to change or modify something you just built, you will spend a good amount of time cursing your past self because you are not able to comprehend what on earth you were thinking.
This article aims to help with that. While nobody is clairvoyant, there are some very easy things you can do today to get your future self to say, “Thankfully I had some foresight!” As a nice side effect, you’re also going to get some love from future colleagues or any other people who may have to modify the app you wrote.
Let’s dive in.
Code Best Practices
If you routinely write code, you know how easy it can be to just “bang something out” and forget about it. The problem is, code rarely stays intact forever. It is true that nobody has a crystal ball but there are a few things you can do to make your life easier in the future. Here are a few ideas to consider.
Comments
Why don’t more devs comment their code? It can be infuriating when you are tasked to edit code written long ago (or by somebody else) and you see it’s 150 lines of gibberish without a single // in sight. Comments don’t have to be long-winded; a little goes a long way. You can go full JavaDocs on every class or method, or you can simply leave a line or two saying things like, “This method will transform X into Z so that we can do Y later” or “I am looping here so I can save the query in the next method.” This is especially important if you’re doing something counter-intuitive or an anti-pattern, but you’re doing it for a reason. You should explain, in excruciating detail, what it is that you are doing and why. That way, you won’t give anyone the chance to say, “What the heck were they thinking?”
What you know today may not be remembered or known tomorrow. You’ll see this concept repeated throughout this article because planning for the future can ease your frustrations next month, as well as in the years to come.
Proper Naming
I know, nobody likes to be super detailed with their comments – it’s boring. Do you know how you can reduce the need for comments? Make sure your variables, methods, and classes are named properly. If you create a map of accounts where the key is the AccountNumber, call it accountsByAccountNumber, not accs. If you have a method to process Widgets and sort them by type, the method should be called sortWidgetsbyType and not process.
This is important because it helps your code be self commenting. We’ve all seen plenty of code with variable names like a, ct, or prc. Understanding what something does or contains by simply looking at it makes it so much easier to get the context and speeds up the process of refactoring or improving code.
Build for Reusability
There is an old developer adage that says “if you did something three times, it is time to abstract it into a function.” This is certainly a good principle. Imagine if you don’t follow it and then somebody requests you make a change in that logic…. And it has to be applied everywhere. It would be brutal to be the person stuck tracking all of that down. Even worse, it increases the chances of missing one instance and introducing bugs in the process.
Sometimes moving code into a common function is a pain, but in many cases, a penny spent now saves a dollar later. Be compassionate to your future self.
Test Code
You might be thinking to yourself, “Wait, why would test code make my life easier later?” Well, it’s simple. Good test code will make your life easier later. That means asserting all kinds of things that your code does and making sure that your outcomes are covered. That way, if you ever make a change that breaks something else or you make changes in two pieces of code but forget a third (see the previous section), then your test code will be there to save your rear-end. Test code, when done correctly, is a lifesaver for refactors because you can minimize the number of regressions and speed up your work.
Consistency
If your code “looks and feels” the same throughout the application, you’ll be able to recognize what it does much faster. You can understand code written with consistency much better than when it’s a hodgepodge of styles. I always tell my teams that the highest praise I can give them is when I can’t tell who wrote a specific piece of code. That means that I can go and modify anybody’s code because it will feel as if I wrote it.
Patterns
Ahhh, Patterns. Devs have a love-hate relationship with Patterns. I won’t go into every single reason why this is good, but let me leave a tidbit. Have you ever needed to change a field in a query from xx__c to yy__c only to find out you have to change 15 different classes? Or you forgot to check for CRUD/FLS and had to track down every DML statement in a project? What if your queries were all neatly put together in a selector class? Or all your DMLs in a service class?
Patterns are there not just to make people sound smart or to increase the number of lines of code you write. Learn them and use them. There is one for every style.
Platform Best Practices
There are also several things you can do now from a platform perspective to make things easier for your future self.
API Names and Labels
Ever wondered why a field is called ThingOneGoesHere__c when in reality it stores ThingTwo__c? Or why it’s called Type__c but there are three different things that could mean Type?
It’s important to make sure your fields (like your properties and objects above) really describe what they store. You have 40 characters – use them! For example, if you have a lookup, try to name that lookup after the object that it points to, unless you have more than one pointing to the same object. Don’t abbreviate unless you have to. And, most importantly, keep your labels in sync with the API name.
Now, we all know that sometimes you don’t have a choice but to change a Field Label, and keeping the API field in sync could be really hard if there is code involved. In those cases, you better make sure that your field description is very clear. And on that note…
Descriptions and Help Text
Descriptions and Help Text are there for a reason. Help Text is for the user to know what to do in a given field and it is essential. However, you should remember that you cannot update a managed field’s Help Text once it’s been installed. While you can package the updated text, existing subscribers will not see it.
That’s why descriptions are so important. Not only are they updateable, but they are also admin only. That means you can be really verbose and clarify what the field is supposed to have and how it’ll be used – especially if the name is counterintuitive or you were forced to make the Label diverge from the API Name. The more clarity here, the better. Nobody has ever complained that a field’s description was too clear.
Sub Flows
Ah, Flow Art… We love to hate you. We have all seen those flows with 350 nodes split all over the place and they do look pretty. But what works for Jackson Pollock probably doesn’t work for Salesforce professionals. Those flows may work sometimes but can you imagine making a tweak anywhere? Your company better hope you never win the lottery and retire to Hawaii since no one but the author will ever be able to figure it out.
Yes, proper names and descriptions will help, but flows are notorious for how hard they are to understand after the fact. Do yourself a favor and break down the flow into smaller pieces. Group associated functionality together and make a subflow out of it, even if it’s not reusable. It’s a lot easier to track down one out of 30 nodes than one out of 300.
If you think you’ve made really cool flow “art”, chances are you’ve done it wrong.
Secure Properly
I know you’re probably wondering what security has to do with maintaining code, but you can never overestimate security. Don’t forget the number one rule of security: Give people only what they need. Why do I mention it here? Because it’s much easier to open up access than it is to close it. If you make an object “Private” and then open it up with a few sharing rules, you can always add more or tweak the ones you have. But if you start with “Public Read Write”, the amount of work you’ll have to do to re-test everything when you go back to “Private” is not fun at all, especially if you have integrations. Give your future self some help and, when possible, lock things down as much as it makes sense.
Summary
My hope is that next time you’re writing or building something new, you will remember the advice in this post. Apps have a way of persisting longer than intended, especially those designed to be “temporary”. Building in such a way that makes your code easy to understand makes maintenance inherently easier and less expensive, freeing you (and others) to focus on the more exciting aspects of your work. If you follow this advice, one day you will wish you had a time machine so that you could travel back in time to thank your past self for setting you up for success.
Companies build their Salesforce businesses better with CodeScience. It is our mission that no ISV navigates Salesforce alone. If you’re looking for guidance on your product, help supporting your customers, or just need to ask an expert, get in touch today!