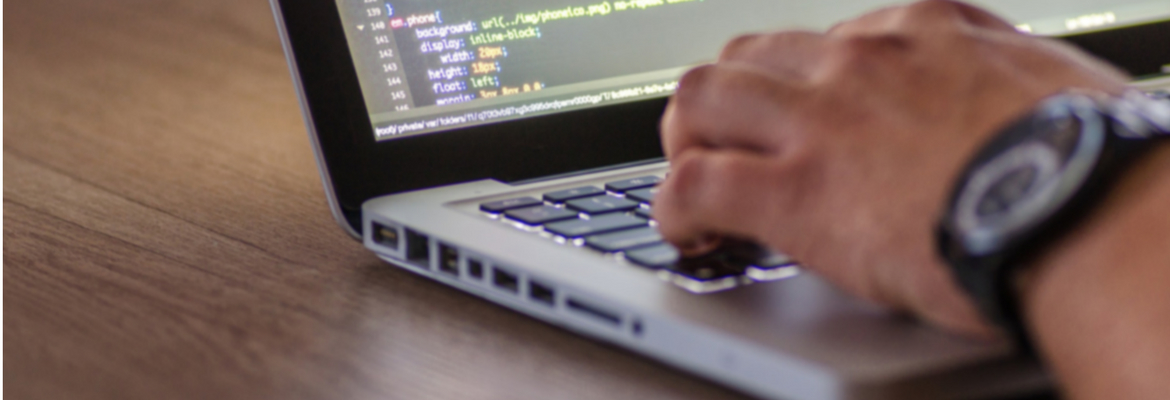
Backend development is the most important component to building a Salesforce application. If code contains mistakes, it can compromise security, function, adoption and revenue — so it’s crucial that we test our app effectively prior to launch. For one of our recent projects at CodeScience, the team sought a way to test a focus area during development by retrieving data from sObjects and the client’s portal, as well as updating Salesforce’s custom objects. This area needed to be tested and functional before the UI was finished.
How can this backend implementation be tested? Do we want to skip testing until the UI is complete? My answer is “No”: Unless there is a purely technical aspect, it’s not a good idea to skip testing.
Since we needed to test ahead of schedule, our team set out to build a framework for closed-loop, efficient testing for QA. This process led to discussions on different testing approaches, with our team weighing the merits of testing through the developer console using SOQL editor or by writing anonymous Apex Code.
We instead ended up creating a Test Harness page to test the back-end — here’s why and how we got there.
Why Test Harness?
During backend implementation, one option is to test the service using Developer Console. Test results are relatively visible, but maintaining all the test scripts or SOQL scripts, demoing completed work, and showing the test results using developer console was quite challenging. So that wouldn’t work well enough for our standards.
The results of coded data retrieval are often poorly formatted and can be confusing, especially to the tester. Most testers cannot follow the anonymous Apex code we’re creating for tests. We wanted the ability to let QA test the backend using a user interface.
That’s why we needed a test page for backend services where the test results can be presented and documented. Our developer, Bobby Tamburrino, came up with an approach of using Visualforce (VF) pages to create a page where the test scripts written to test backend services are called.
With a click of mouse on a custom user-friendly screen, instead of using anonymous Apex code in the Developer Console, clients can test and view results and our QA team can test/document them.
What is Visualforce?
Visualforce(VF) is the technology for developing custom pages in Salesforce. VF provides input components like check boxes, lists, text areas. etc. It also provides the ability to use buttons, which can trigger actions like saving, retrieving, deleting, or updating data. After those actions happen, it’s possible for data to display on the same page. You can learn more about VF here.
Simple Scenario -Test/Display results in VF page
Here’s an example scenario showing how this works:
Retrieve all the account records with the user given search string as the input, and sort them by ‘Industry’ in ascending order.
Step 1: Create a form
Step 2: Add an input textarea field with label = Account
Step 3: Add a field to enter the condition for sorting
Step 4: Add a button with label = Get Accounts; action should fetch all the records with a given search string
Step 5: Add an output section to display results
<apex:page controller="AccountSearch"> <apex:form > <apex:pageMessages ></apex:pageMessages> <apex:pageBlock title="Account Search" > <apex:inputText value="{!searchString}"/> <apex:inputText value="{!sortField}"/> <apex:commandButton action="{!searchAccounts}" value="Search" reRender="messages" /> </apex:pageBlock> <apex:pageBlock title="Account Results"> <apex:pageBlockTable value="{!records}" var="record"> <apex:column > <apex:facet name="header">Account Name</apex:facet> <apex:outputText value="{!record.Name}"/> </apex:column> <apex:column > <apex:facet name="header">Account Number</apex:facet> <apex:outputText value="{!record.AccountNumber}"/> </apex:column> </apex:pageBlockTable> </apex:pageBlock> </apex:form > </apex:page > //Account Search Controller public with sharing class AccountSearch{ public String searchString {get;set;} public String sortField {get;set;} public List<Account> records {get; set;} public searchAccounts() string strLikeString = '%'+searchString+'%'; string sortString = '%'+sortField+'%'; string strSOQL = 'select AccountNumber,Name from Account where name LIKE: String.escapeSingleQuotes(strLikeString) order by :String.escapeSingleQuotes (sortString) asc'; records = database.query(strSOQL); } }
Conclusion
Thanks again to CodeScience Developer and Team Lead Bobby Tamburrino, who came up with this solution. This approach helped us test the back-end implementation in an effective and timely manner.
The Test Harness behaved just like any other user interface, allowing our client and QA team to enter inputs and see outputs on the same page with the click of a button. The user doesn’t see the test scripts that are called to retrieve the results. With this approach, we created two different Test Harness pages for the client to use and verify that work was done the right way — ensuring a high-quality build to hit the ground running.
Want swift app development that you’re able to test, or need a custom build for something great? You’re in the right spot. Just let us know what you’re working on and we’ll be in touch!